Decryption of AsyncRAT config strings with CyberChef
Yesterday, as a part of a challenge in one CTF competition, I had to analyze a modified sample of AsyncRAT. I will try to avoid any spoilers, however, I wanted to decode and decrypt strings from AsyncRAT configuration settings.
AsyncRAT is written in C#, and there are various variants and clones in the wild, such as DcRat or VenomRAT. Some samples are almost not obfuscated (except the encryption of the configuration), some are lightly obfuscated with just renamed methods and classes. But there are also samples which are obfuscated more, for example packed into other trojans executables or into PowerShell scripts.
During my analysis, I extracted similar DcRat sample, decompiled it and as an exercise, I created CyberChef recipe for decrypting the strings from the config of the sample. Then, I found several other AsyncRAT samples and veryfied that my CyberChef recipe is usable for their analysis, too. Of course, it was just an exercise, since there are several config extractors available online.
Decompilation of AsyncRAT
All screenshots and examples are from the recent sample of AsyncRAT, available on Any.Run and VirusTotal.
I used the ILSpy Decompiler, or its command-line version ILSpyCmd. After a decompilation of the AsyncRAT, there is a class called Client.Settings
(if the code is not obfuscated). If the methods are renamed, you can recognize this class based on several Base64-encoded strings, as you can see on Figure 1. We can also use command
ilspycmd -t Client.Settings AsyncRAT_sample
for decompile the Settings class only.
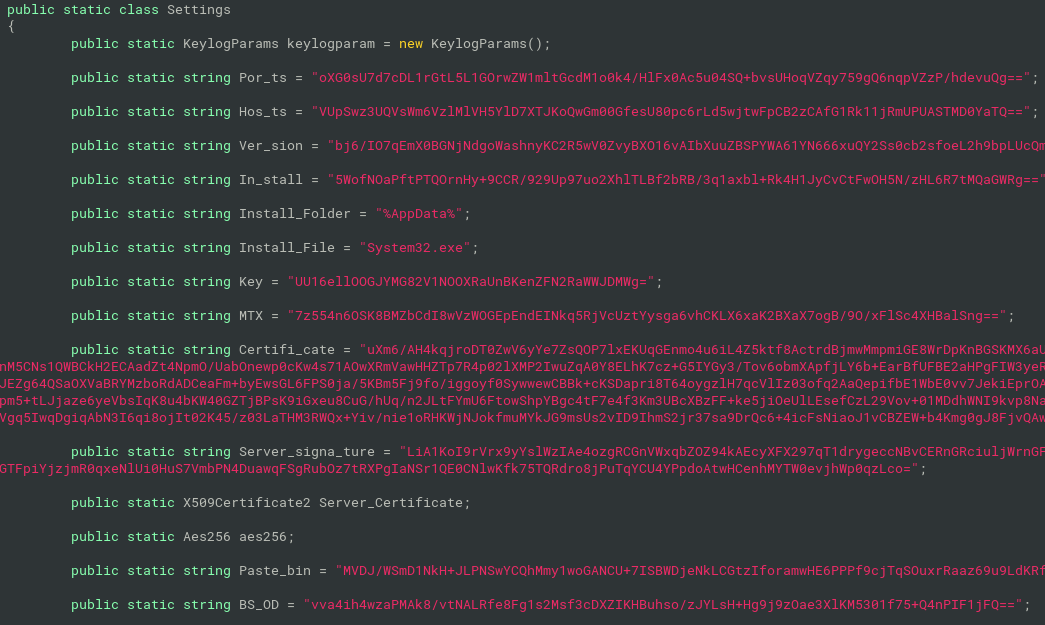
Then, those strings are decrypted using AES-256 with the key based on the Base64-decoded Key value from Settings:
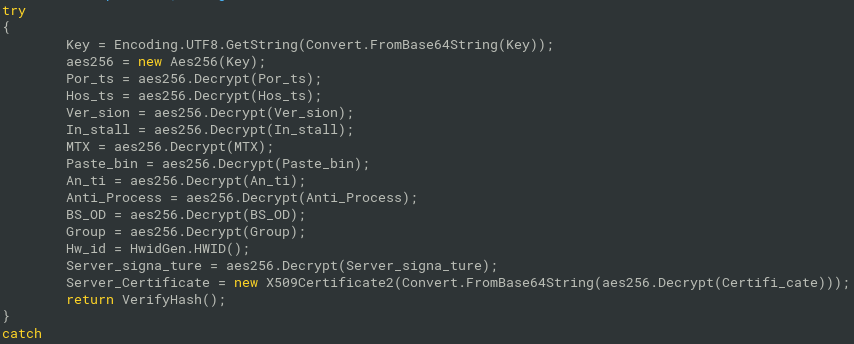
The decoded Key value is used as a masterKey for PBKDF2 (function Rfc2898DeriveBytes()
) with Salt
defined in the properties of Client.Algorithm.Aes256
class (“VenomRATByVenom” in this case)” and 50000 iterations:
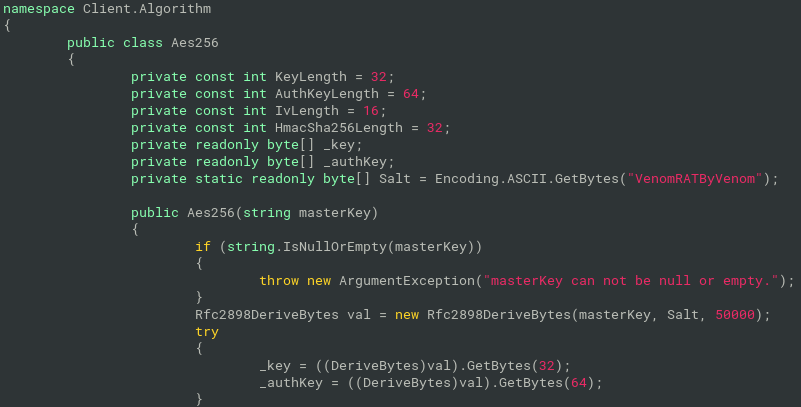
Finally, the encrypted string messages are firstly decoded from Base64 and then decrypted with Aes-256 class. However, their internal format is the following:
HMAC[32] + IV[16] + CipherText
where HMAC is 32-bytes long SHA256-based message authentication code and IV is 16-bytes long Initialization vector used as additional parameter for AES decryption/encryption. After HMAC and IV, there is the encrypted plaintext itself. So, for the decryption, we could skip the HMAC, extract IV and used IV together with derived key for AES decryption, with the additional parameters from the Figure 4. Key size is 256, block size 128, mode is CBC and padding is PKCS7.
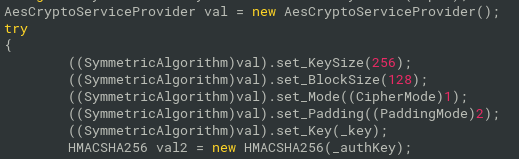
Decryption with CyberChef
Now we are theoretically prepared. We already know how the config strings are encrypted and encoded, how is derived key for AES and what parameters of AES decryption are used. It is time to play with CyberChef and reimplement the decryption routine in this cyber swiss-army knife.
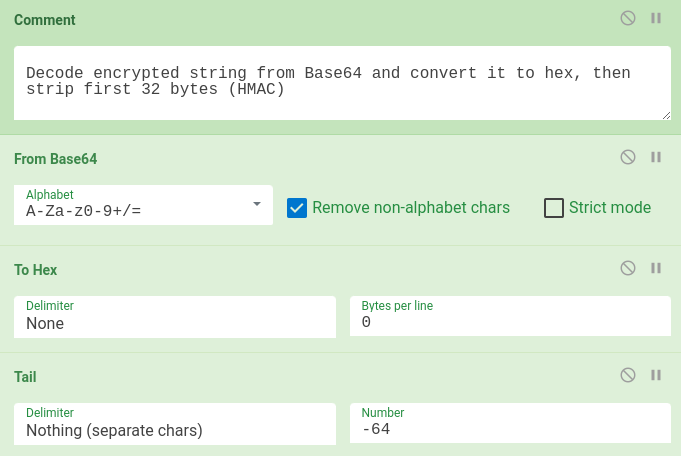
The first task is to extract IV and CipherText from encrypted message. After Base64 decoding, we do not need to verify HMAC, so we can skip the first 32 bytes of encrypted message (64 chars in hex-encoded message) with tail
(see Figure 5). Then, the next 16 bytes contain IV. We can extract them with regular expression and store them into CyberChef register variable $R0
(see Figure 6).
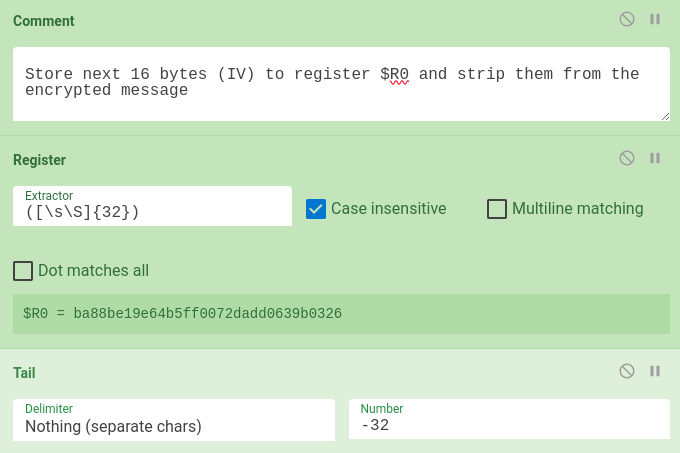
Before we switch to derivation of the key with PBKDF2, we need to preserve the ciphertext from current input. Again, we can store the input into the next register $R2
. Now, we can derive the key for AES with PBKDF2 - in the following operation, there are used parameters Master Key (or Passphrase) from Client.Settings
and Salt from Client.Algorithm.Aes256
. We will remember the derived key in the next register $R2
.
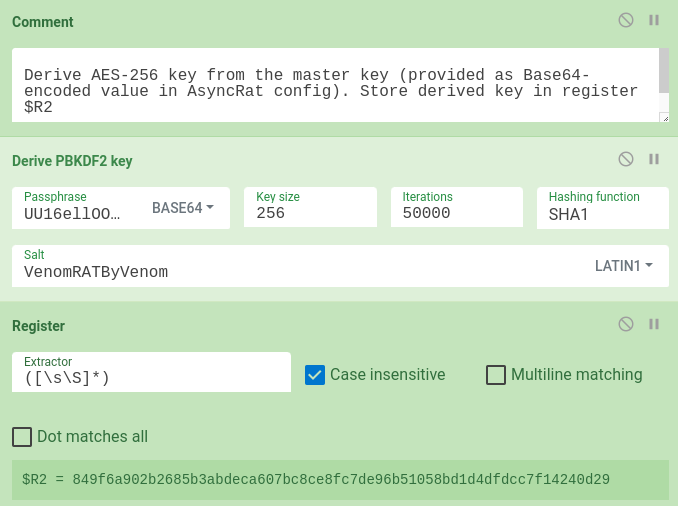
Finally, we will bring back the remembered cipertext from $R1
to the pipeline and we can decrypt it with the AES with key from $R2
and IV from $R0
.
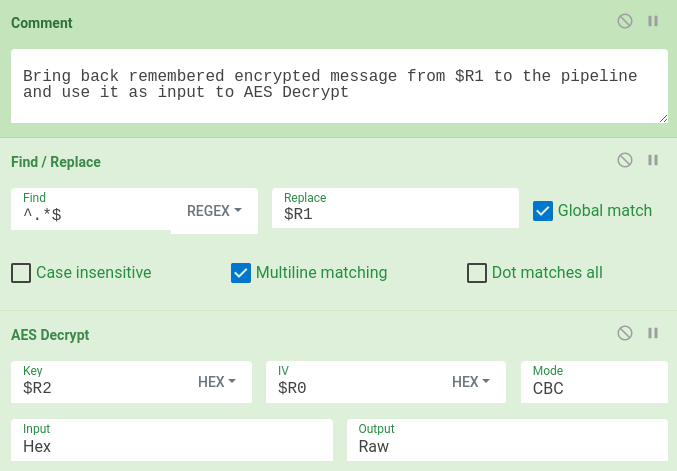
In AsyncRAT/DcRat/VenomRAT settings, there is also embedded Certificate. If we want to decrypt and parse this certificate, we can add one additional step of Parse X.509 certificate
:
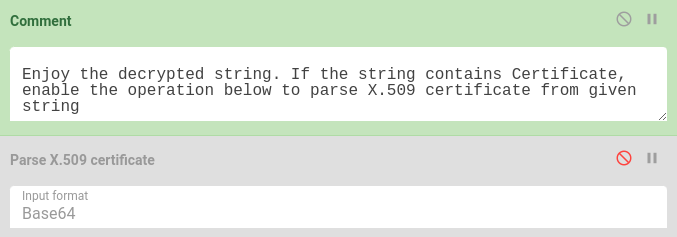
Conclusion
For easy of use, the CyberChef recipe from previous section is available online. And there is also the same recipe with an example input, too.
It was interesting exercise for me to play with CyberChef and its registers.
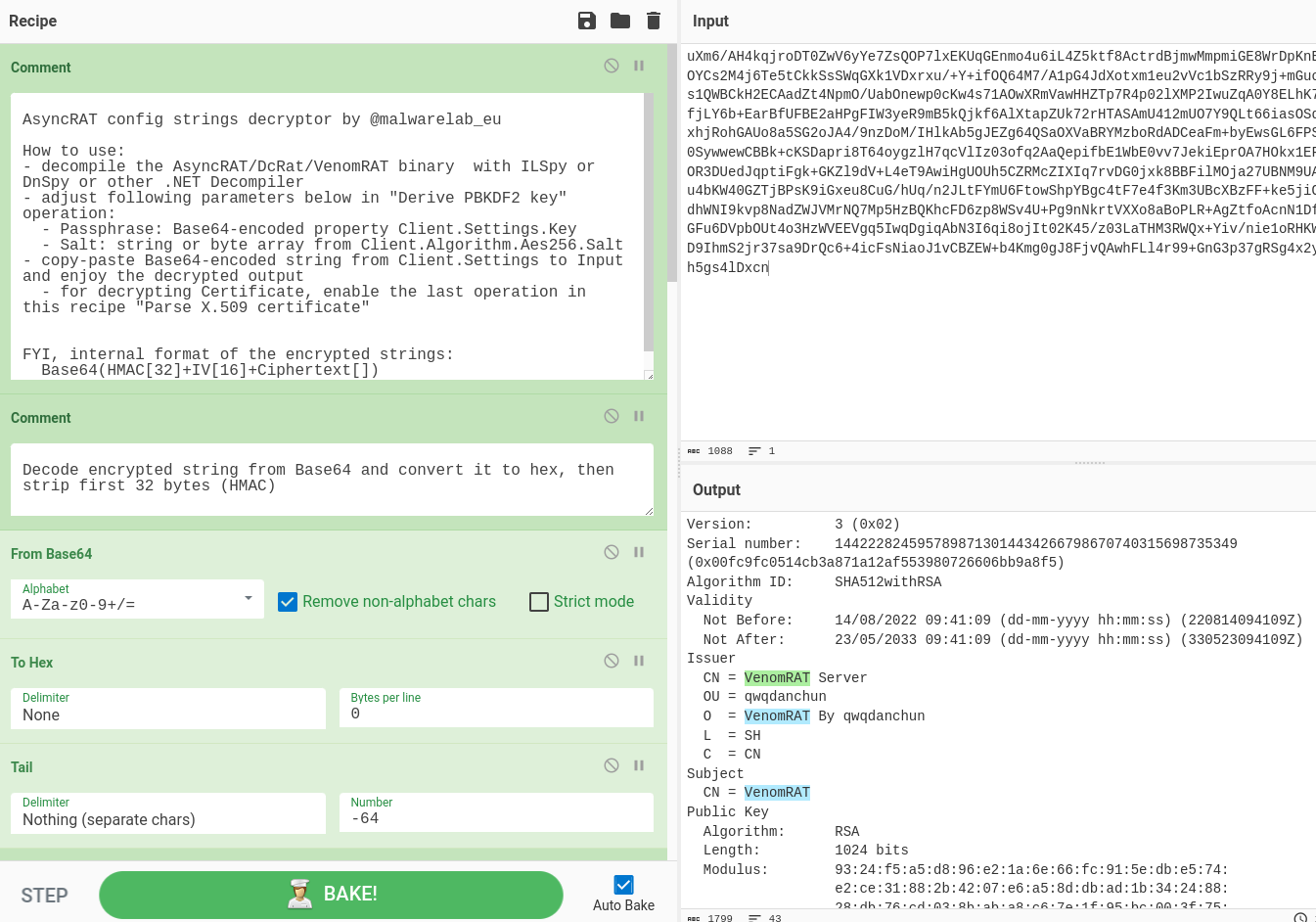